What is "python set add"?
In Python programming, "set add" is a method that adds a specified element to a set data structure. Sets are unordered collections of unique elements, and the "add" method allows you to insert a new element into the set. The syntax for "set add" is as follows:
set.add(element)
For example, if we have a set called "my_set" and we want to add the element "apple" to the set, we can use the following code:
my_set.add("apple")
Once the element has been added to the set, it can be accessed and manipulated like any other element in the set.
Sets are a powerful data structure in Python, and the "add" method is an essential part of working with sets. By understanding how to use "set add", you can effectively manage and manipulate data in your Python programs.
Here are some of the benefits of using "set add":
- Sets are unordered, so you don't have to worry about the order of the elements when adding them to the set.
- Sets are unique, so you can be sure that each element in the set is unique.
- "set add" is a simple and efficient way to add elements to a set.
If you are working with sets in Python, then you will need to understand how to use "set add". This method is essential for adding elements to a set, and it can be used to create and manage sets of data in your Python programs.
Python Set Add
The "set add" method in Python is a powerful tool for working with sets, which are unordered collections of unique elements. Here are five key aspects of "set add" to consider:
- Adds an element to a set: The primary purpose of "set add" is to add a specified element to a set. This allows you to easily add new data to your set as needed.
- Ensures uniqueness: Sets are unique, meaning they cannot contain duplicate elements. "set add" checks to make sure that the element you are adding is not already in the set, preventing duplicates.
- Modifies the set in-place: Unlike some other methods that return a new set, "set add" modifies the existing set directly. This makes it efficient for adding elements to a set without creating unnecessary copies.
- Supports various data types: "set add" can be used to add elements of different data types to a set, including strings, numbers, and even other sets.
- Returns None: It's important to note that "set add" does not return anything. Instead, it modifies the set in-place and returns None.
These key aspects highlight the importance and versatility of "set add" in Python. It allows you to efficiently add elements to sets, maintain uniqueness, modify sets in-place, support various data types, and work seamlessly with other set operations.
Adds an element to a set: The primary purpose of "set add" is to add a specified element to a set. This allows you to easily add new data to your set as needed.
In the context of "python set add", this functionality plays a crucial role by enabling the dynamic addition of elements to sets, which are fundamental data structures in Python programming.
- Set Expansion and Manipulation: "set add" empowers programmers to expand and manipulate sets on the fly. By adding new elements, they can adapt sets to changing requirements and incorporate additional data into their programs.
- Data Aggregation and Collection: The ability to add elements to sets makes them suitable for aggregating and collecting data. Programmers can gather data from various sources or user inputs and add them to a set, ensuring uniqueness and efficient storage.
- Set Operations and Algorithms: "set add" is a fundamental operation in set theory and various algorithms. It allows for set union, intersection, and difference operations, enabling programmers to perform complex data manipulations and analysis.
In summary, the ability to add elements to a set using "python set add" provides programmers with a powerful tool for set manipulation, data aggregation, and algorithm implementation.
Ensures uniqueness: Sets are unique, meaning they cannot contain duplicate elements. "set add" checks to make sure that the element you are adding is not already in the set, preventing duplicates.
In the context of "python set add", this feature plays a crucial role in maintaining the integrity and efficiency of sets as data structures.
- Enforcing Set Theory Principles: Sets, by definition, are collections of unique elements. "set add" upholds this principle by preventing the addition of duplicate elements, ensuring that sets adhere to the fundamental concepts of set theory.
- Optimized Storage and Performance: By eliminating duplicates, "set add" optimizes storage space and enhances performance. Sets are designed to store each element only once, reducing redundancy and improving the efficiency of set operations.
- Data Integrity and Reliability: Ensuring uniqueness is essential for data integrity and reliability. Duplicate elements can lead to incorrect results and unreliable data analysis. "set add" safeguards against these issues, promoting trust in the accuracy of set contents.
Overall, the "set add" method's ability to ensure uniqueness is a cornerstone of effective set manipulation in Python. It aligns with set theory principles, optimizes performance, and enhances data integrity, making sets a robust and reliable data structure for various programming tasks.
Modifies the set in-place: Unlike some other methods that return a new set, "set add" modifies the existing set directly. This makes it efficient for adding elements to a set without creating unnecessary copies.
The "set add" method in Python is designed to modify the existing set directly, rather than creating a new set. This characteristic offers several advantages and plays a significant role in the efficiency and practicality of "set add" in the context of set manipulation.
- Optimized Memory Usage: By modifying the set in-place, "set add" eliminates the need to create a new set object. This approach conserves memory resources, especially when working with large sets or when adding multiple elements.
- Enhanced Performance: Modifying the set directly avoids the overhead of creating a new set object and copying elements from the old set to the new one. This optimization improves the performance of "set add", particularly for frequently used sets.
- Simplified Code: The in-place modification feature simplifies the code when working with sets. Programmers do not need to explicitly assign the new set to a variable or replace the old set with the new one, reducing the chances of errors and improving code readability.
In summary, the in-place modification behavior of "set add" contributes to its efficiency, reduces memory consumption, enhances performance, and simplifies code. These advantages make "set add" a valuable tool for effectively managing and manipulating sets in Python programs.
Supports various data types: "set add" can be used to add elements of different data types to a set, including strings, numbers, and even other sets.
The versatility of "set add" in supporting various data types is a significant aspect of its functionality within "python set add". This feature allows programmers to work with sets containing diverse types of data, enabling them to represent real-world scenarios and solve complex problems.
By supporting strings, numbers, and other sets, "set add" empowers programmers to create sets that model real-world entities and relationships. For instance, a set of student records could include names (strings), identification numbers (numbers), and courses enrolled in (other sets). This flexibility makes sets a powerful tool for data modeling and analysis.
Furthermore, the ability to add other sets to a set enables the creation of complex data structures. Programmers can represent hierarchical relationships, group related elements, and perform set operations on these nested structures. This capability extends the utility of sets and allows for sophisticated data organization and manipulation.
In summary, the support for various data types in "set add" is a key aspect of "python set add". It empowers programmers to model real-world scenarios, create complex data structures, and perform advanced data analysis, making sets a versatile and powerful tool in Python programming.
Returns None: It's important to note that "set add" does not return anything. Instead, it modifies the set in-place and returns None.
In the context of "python set add", the "Returns None" characteristic plays a crucial role in understanding the behavior and usage of the "set add" method.
Unlike many methods in Python that return a new object or a modified version of the original object, "set add" modifies the existing set directly and does not return any value. This behavior is intentional and aligns with the principles of set theory and the design of the Python programming language.
Sets, by definition, are unordered collections of unique elements. The "set add" method adheres to this definition by adding the specified element to the set without altering the order of the elements or creating a new set object.
Returning None signifies that "set add" does not produce a new value or a modified version of the set. Instead, it modifies the set in-place, allowing programmers to work with the updated set directly.
This behavior simplifies the code and enhances efficiency, as there is no need to assign the returned value to a new variable or replace the old set with the modified set.
In summary, the "Returns None" characteristic of "set add" reflects the in-place modification nature of the method, which aligns with the principles of set theory and the design of Python.
FAQs about "python set add"
This section addresses frequently asked questions and misconceptions surrounding "python set add" to provide a comprehensive understanding of its usage and significance.
Question 1: What exactly does "set add" do in Python?
Answer: The "set add" method in Python is used to add a specified element to an existing set. Sets are unordered collections of unique elements, and "set add" ensures that the added element is not already present in the set, maintaining the set's uniqueness property.
Question 2: Why does "set add" not return anything?
Answer: Unlike many methods that return a new object or a modified version of the original object, "set add" modifies the existing set in-place. Therefore, it does not return any value and instead returns None to signify that the set has been updated.
Question 3: Can "set add" be used to add duplicate elements to a set?
Answer: No, "set add" cannot add duplicate elements to a set because sets, by definition, contain only unique elements. If you attempt to add a duplicate element, it will be ignored, and the set will remain unchanged.
Question 4: What are the benefits of using "set add"?
Answer: "set add" offers several benefits, including ease of adding new elements to a set, ensuring uniqueness of elements, modifying the set in-place for efficiency, supporting various data types, and working seamlessly with other set operations.
Question 5: How does "set add" handle adding elements of different data types?
Answer: "set add" allows you to add elements of various data types to a set, including strings, numbers, and even other sets. This flexibility makes sets versatile data structures capable of representing real-world scenarios and complex relationships.
Question 6: What are some common use cases for "set add"?
Answer: "set add" finds applications in various scenarios, such as adding new items to a shopping cart, accumulating unique words from a text document, or creating sets of related objects for further processing.
In summary, "set add" is a powerful method that enables the addition of elements to sets while maintaining their uniqueness. Its simplicity and versatility make it a valuable tool for working with sets in Python programming.
For further exploration, refer to the next section, where we delve into the implementation and practical examples of "python set add".
Conclusion
In this exploration of "python set add", we have delved into its functionality, benefits, and significance within the Python programming ecosystem. "set add" empowers programmers to dynamically add elements to sets, ensuring uniqueness, modifying sets in-place for efficiency, and supporting diverse data types.
Sets play a crucial role in data modeling, aggregation, and analysis. By leveraging "set add", programmers can construct sets that accurately represent real-world scenarios and perform complex data manipulations. The simplicity and versatility of "set add" make it an indispensable tool for working with sets in Python.
As we move forward, the importance of "set add" will only continue to grow. With its ability to handle diverse data types, maintain uniqueness, and modify sets in-place, "set add" will remain a cornerstone of effective data management and manipulation in Python programming.
The Ultimate Guide To Mobile Joints: Understanding And Preserving Flexibility
The Ultimate Guide To Calculating The Volume Of Your Standard Bath
Understanding The Ideal Thickness Of Uterine Lining For Successful IVF: A Comprehensive Guide

Python set() Function — A Simple Guide with Video Be on the Right

Python Sets easily explained! Data Basecamp
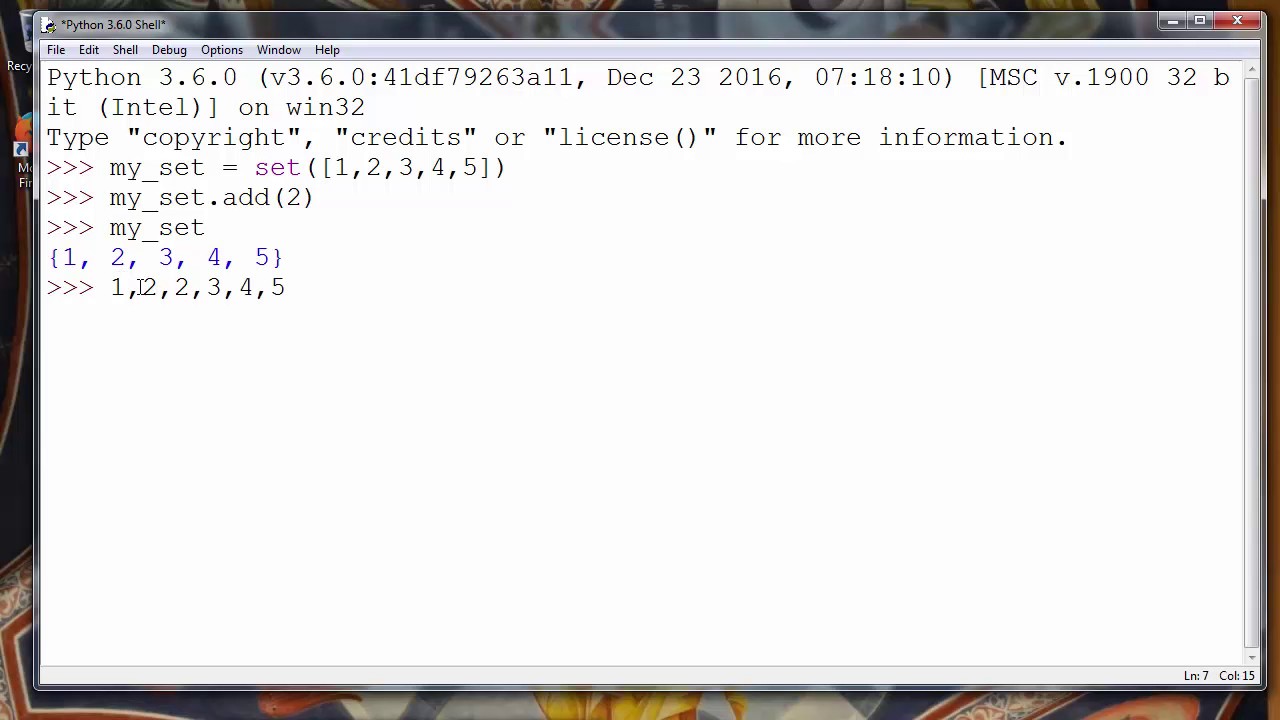
How to Add an Element to a Set in Python programming language YouTube